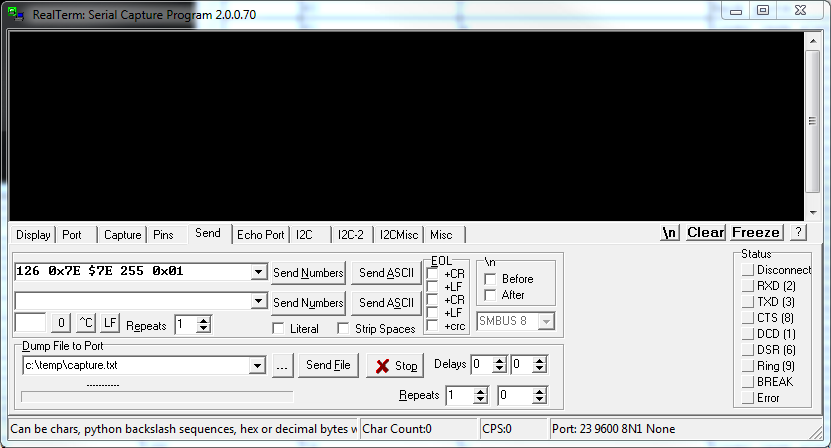
Python Serial Port Example Windows Batch
I would like to read from the USB serial port from time to time in a loop. I can open the port and read data with: import serial ser = serial.Serial('/dev/ttyACM0', 9600) while 1: ser.readline() An Arduino Uno is connected to the USB port of the RPi.
The Arduino acts as a sensor and it will constantly produce readings. I need some help in adding timing features to the above code. I want to open the port and read from it for a certain period of time. After that period of time, the port closes and the received data will be analysed. After a pause of several minutes, the port will reopen and the RPi will read data from it again.
This continues in a loop. Any help is much appreciated.
'Tutorial for programming the serial port on Windows using API and serially communicating with an external microntroller board like Launchpad.' 'Compiling the serial port communication program program using MinGW on Windows 7. Nov 5, 2014 - python -c 'import serial.tools.list_ports as ls;print list(ls.comports())'. So I can see anything plugged in. Is a windows command line utility to get system information. If your serial port is virtual created by some driver through USB connection, use this example to get details about these serial ports.
All you would need to add, aside from closing the port when you're done;), is import time and then use: import serial, time ser = serial.Serial('/dev/ttyACM0', 9600) while 1: serial_line = ser.readline() print(serial_line) # If using Python 2.x use: print serial_line # Do some other work on the data time.sleep(300) # sleep 5 minutes # Loop restarts once the sleep is finished ser.close() # Only executes once the loop exits I don't know if pySerial is buffered (data sent while sleeping is stored or simply dropped), but I usually prefer to use a generator, if you don't explicitly need to wait. They seem a bit more flexible (in my opinion): def serial_data(port, baudrate) ser = serial.Serial(port, baudrate) while True: yield ser.readline() ser.close() for line in serial_data('/dev/ttyACM0', 9600): [.transform data.] You might also be able to use the with syntax instead of the while, but I'm not too sure how that'd work with pySerial. I was just editing my comment to clarify, but locked after 5 minutes:/ The ser.close() never runs until the while loop is done (which doesn't really ever occur since it's while 1/ while true), so the serial port isn't actually closed between readline() calls. Since simply having a pySerial port open isn't blocking, that shouldn't be an issue. If the while loop only ran x number of times, and then you wanted to work on that the port again, just leave the port open until done (move ser.close() after all code that interacts with the port).
Elegiac cycle brad mehldau transcription pdf files. Elegiac Cycle is a solo studio album by American pianist Brad Mehldau. The album was issued in 1999 by Warner Bros. Produced by Melhdau himself. Looking Back on Elegiac Cycle This essay is an excerpt from an extended interview conducted several years ago that appeared in the introductory material to the transcription book of Brad's record from 1999, Elegiac Cycle. Transcriptions & Brad Mehldau March 01, 2010 / Andrei Strizek I got an email about 2 weeks ago from someone in Europe (I can't remember where - Denmark, I think) asking me for a copy of my transcription of Brad Mehldau's Goodbye Storyteller (for Fred Myrow) (listen to the piece below), a solo piano piece from his incredible album Elegiac Cycle. Brad Mehldau Collection - Six Transcriptions.pdf. Brad Mehldau Collection - Six Transcriptions.pdf. Brad-Mehldau Exit Music. Brad Mehldau Metheny Mehldau Quartet. Brad Mehldau Elegiac Cycle. User Ratings (0) Your Rating. It's Brad Mehldau's 'Elegiac Cycle'. Also a transcription by jazz pianist Philippe Andre is available from Amazon which will add to anyone's. Welcome to 'Brad Mehldau. Brad Mehldau – Transcriptions Part 2. Elegiac Cycle Brad Mehldau Transcription Pdf Reader. Elegiac Cycle - Wikipedia, the free encyclopedia. Elegiac Cycle is a 1. Brad Mehldau. The album was issued in 1.
– Apr 30 '14 at 19:01 •.
The pre-shared key is known as the 'Shared Secret' within the settings. As soon as you change this key all of your existing clients will be unable to connect as they will all We link ours straight through to the AD user account and there is good sonicwall documentation out there showing how to do this. Enabling a Pre-Shared Key VPN Connection. SonicWALL Global VPN Client 1.0.0.0 - 1.0.0.9 User’s Guide Page 5 Installing the SonicWALL Global VPN Client. You can't change the 'pre-shared' key on the client side - the clue is in the name, it's included with the policy file which you distribute to clients. How to crack pre shared key sonicwall.
I need to access the serial port via python on a windows machine. Reading on the web, there are three solutions: pyserial, siomodule and USPP. Pyserial seems to be the best option since the other two are tested with Windows 95 and older versions of python.
Would you agree with this or have I missed an option? Can anyone provide me an example of how to access the serial port with pyserial? Thanks George T. From http Mon Feb 6 21: From: http (Paul Rubin) Date: 06 Feb 2006 12:04:21 -0800 Subject: Webmail with Python References: Message-ID: Thomas Guettler writes. Roel Schroeven I hadn't even heard of siomodule and USPP; pyserial is what I use when I need to read/write from/to the serial port.
It's quite simple; there are a number of examples on pyserial's website. Here's a small quick and dirty script that reads data from a serial port and broadcasts it as UDP over the network to make the incoming data available to other computers in the office: import socket import sys import serial ser = serial.Serial('COM1', 38400, timeout=1) sock = socket.socket(socket.AF_INET. Can anyone provide me an example of how to access the serial port with pyserial?It's quite simple; there are a number of examples on pyserial's website. Here's a small quick and dirty script that reads data from a serial port and broadcasts it as UDP over the network to make the incoming data available to other computers in the office: import socket import sys import serial ser = serial.Serial('COM1', 38400, timeout=1) sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) sock.setsockopt(socket.SOL_SOCKET, socket.SO_BROADCAST, 1) while True: msg = ser.readline() sock.sendto(msg, (', 5000)) sys.stdout.write(msg) Basically you can use the objects like other file-like objects. On 2006-02-06, George T. Wrote: I need to access the serial port via python on a windows machine.